Working with Time Off
This guide walks through how to list, create, update, and cancel time off requests using the Remote API. Each time off must belong to a type, and all available types are listed in the “Time off types” section below. Additionally, a time off has a status attribute that can assume one out of the six statuses listed in the “Time off status” section.
Definitions
Time off types
The list of available time off types is dynamic as new types might be added in the future. For the complete list of types and their descriptions, choose one returned by the List Time Off Types endpoint.
Below there's an example of how to fetch the list of available types and their descriptions:
1curl --location --request GET \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff/types
Time off status
Here is the list of available time off statuses:
approved
: The employee time off request was accepted by the employer.taken
: An approved request has been used.declined
: The employee time off request was declined by the employer.requested
: The initial state of a time-off request. This means the request is waiting for employer approval.cancel requested
: The employee asked the employer to cancel a past time off.canceled
: Means that the request was canceled.
The approver_id
field
The approver_id
field must match the id
of a user in the Remote Platform that has permission to approve time off requests. Available users can be found fetching the List Company Manager endpoint.
1curl --location --request GET \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/company-managers
The timeoff_days
field
The start_date
and end_date
define the duration of the leave. If the leave takes only one day, then both values should be the same.
Regardless of that, the timeoff_days
array must contain one entry per day, even when creating time off for a single day. For example, in a leave from Nov 3 to 4 of 2022, it requires a structure such as:
1[2 {"day": "2022-11-03", "hours": 8},3 {"day": "2022-11-04", "hours": 8}4]
The number of hours must be an integer between 0 and 8, meaning the number of hours not worked on the referred day.
If the leave period has weekends or other non-workable days, the number of hours must be 0. For example, a leave from Nov 3 to Nov 7 of 2022, would require the following structure:
1[2 {"day": "2022-11-03", "hours": 8},3 {"day": "2022-11-04", "hours": 8},4 {"day": "2022-11-05", "hours": 0}, // Saturday5 {"day": "2022-11-06", "hours": 0}, // Sunday6 {"day": "2022-11-07", "hours": 8}7]
This system supports partial days off. For example, a half day can be requested by using the value 4
in the hours
field.
Operations
Listing employee's time off
The following request will return a paginated response of time off for all employments:
1curl --location --request GET \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff
It’s possible to filter the list of time off by employment, by using employment_id
:
1curl --location --request GET \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff?employment_id=f73b4156-64ba-444f-895b-9da0fca9f303
It's also possible to filter results by status
. For instance, for listing only approved time-offs:
1curl --location --request GET \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff?status=approved
Creating a time off
Currently, the Remote API only supports creating already-approved time off. Here is an example of a valid creation payload:
1curl --location --request POST \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff \5 --data '{6 "start_date": "2022-10-31",7 "end_date": "2022-11-02",8 "employment_id": "5509b6b0-5a0a-11ed-a6f6-c38dbde70e6f",9 "timeoff_days": [10 {day: "2022-10-31", hours: 8},11 {day: "2022-11-01", hours: 8},12 {day: "2022-11-02", hours: 8}13 ],14 "timeoff_type": "paid_time_off",15 "timezone": "Etc/UTC",16 "status": "approved",17 "approver_id": "e55eedbe-7c17-46e4-bdd2-2b63d56eb2fc",18 "approved_at": "2022-10-27T15:03:23Z"19}'
Attaching a document
The Remote API allows attaching a Time off document. The document
field requires two nested fields: content
and name
.
The content
should contain the file's binary encoded using the Base64. The name represents the filename, which should only contain the base name — not the full path. Below there is an example of a request with an attached time off document.
1curl --location --request POST \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff \5 --data '{6 "start_date": "2022-10-31",7 "end_date": "2022-11-02",8 "employment_id": "5509b6b0-5a0a-11ed-a6f6-c38dbde70e6f",9 "timeoff_days": [10 {day: "2022-10-31", hours: 8},11 {day: "2022-11-01", hours: 8},12 {day: "2022-11-02", hours: 8}13 ],14 "timeoff_type": "sick_leave",15 "timezone": "Etc/UTC",16 "status": "approved",17 "approver_id": "e55eedbe-7c17-46e4-bdd2-2b63d56eb2fc",18 "approved_at": "2022-10-27T15:03:23Z",19 "document": {20 "content": "JVBERi0xLjMKJcTl8uXrp/Og0MTGCjMgMCBvYmoKPDwgL0Zpb...",21 "name": "certification_of_illness.pdf"22 }23}'
Updating an approved time off
Currently, the API only supports updating an approved time off, and always requires a reason for the change. The reason for the change should be put in the edit_reason
field.
1curl --location --request PATCH \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff/daba19dc-61e7-11ed-97bb-5bf1862b6b44 \5 --data '{6 "timeoff_type": "paid_time_off",7 "edit_reason": "Selecting the correct time off type."8}'
start_date
or end_date
field is changed, it's crucial to reflect the changes in the timeoff_days
field as well, as described in the section “The timeoff_days
field” above.Cancelling an approved time off
taken
. However, the employee can cancel it using the Remote Platform following these steps. Cancelling an approved time off requires updating the status
to canceled
and providing a cancel_reason
in the same payload. Below there's an example of how to cancel a time off.
1curl --location --request PATCH \2 --header "Authorization: Bearer <your token>" \3 --header "Content-Type: application/json" \4 https://gateway.remote-sandbox.com/v1/timeoff/daba19dc-61e7-11ed-97bb-5bf1862b6b44 \5 --data '{6 "status": "cancelled",7 "cancel_reason": "Not taking this leave anymore."8}'
Webhooks
Time Off Lifecycle and API Flow
Below, you can see some flowcharts regarding webhook events that are triggered in the Time Off lifecycle and in the API flow:
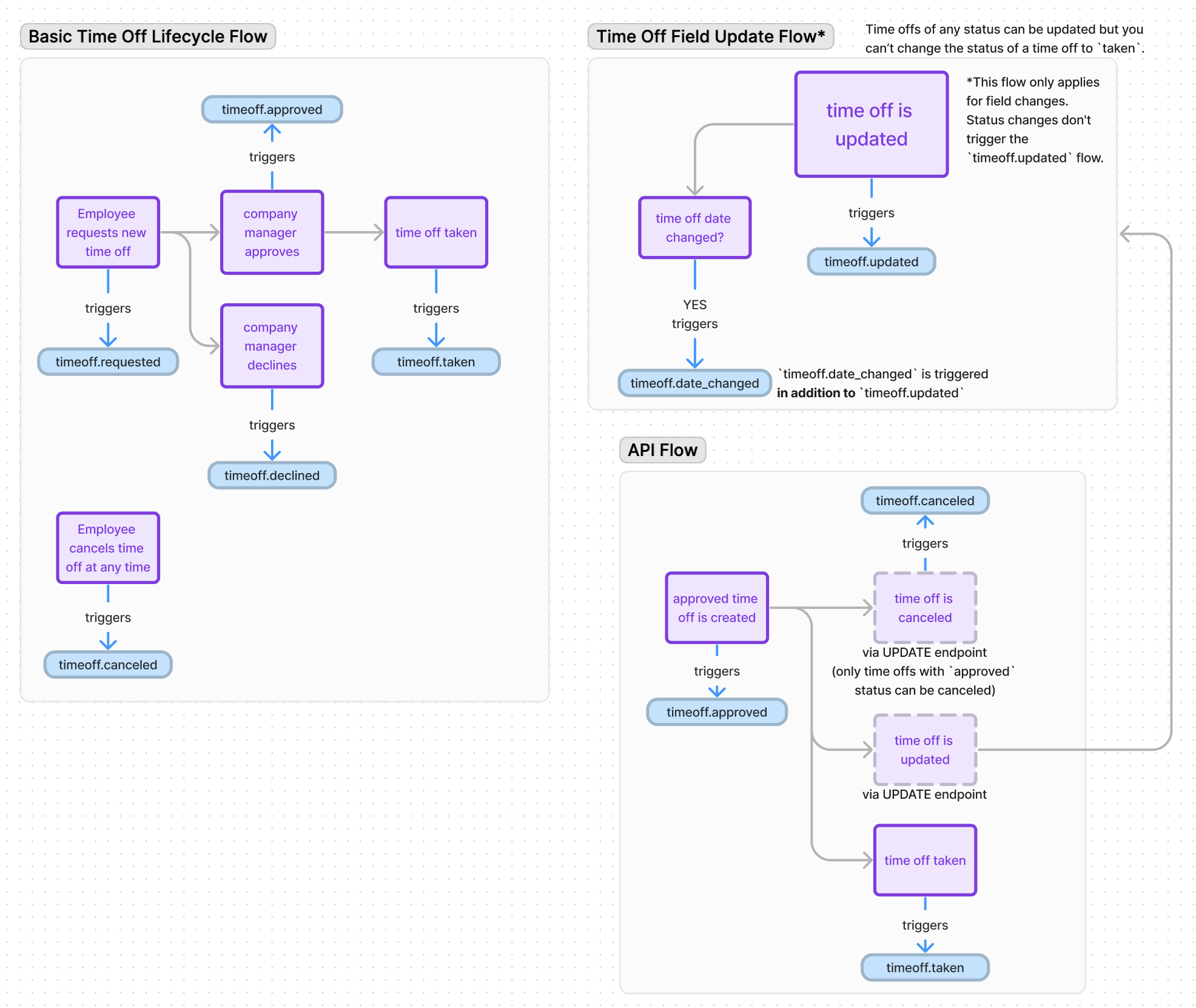
Automatic Holidays
Automatic holidays are national holidays recognized by Remote that are booked automatically for all employees of the country. Here’s the list of the webhook events that can be triggered by this feature:
timeoff.approved
: Since automatic holidays are always created with anapproved
status, the webhook event is triggered at this moment.timeoff.canceled
: Triggered whenever an automatic holiday is removed.timeoff.date_changed
: Whenever a date is recognized by Remote as a national holiday, it is automatically booked for all employees of that country. This can cause a conflict, as an employee could have previously scheduled PTO that includes this date, and now they are entitled to have it as a holiday instead of a PTO. In this case, the conflict is automatically resolved by having the time off hours of that date changed to take the holiday into consideration, which then triggers thetimeoff.date_changed
event.