Money Format
Overview
In the Remote API, you will frequently make use of money values. In this guide, you’ll learn how to interpret them and what caveats to take into account.
In summary, all monetary values that Remote handles have two fraction decimals, which means you must multiply your input value by 100 before sending it to the API. For example, 100.99 EUR needs to be sent as 10099
.
Format
A momentary value is an integer
, which means it does not accept decimals. For that reason, we store monetary values as units that include the two fraction decimals. This format is the same across the API.
Here are a few examples of input value (what users perceive) vs the API value:
Input value | API value |
$ 1.00 | 100 |
$ 10.99 | 1099 |
$ 1500.89 | 150089 |
This means you must multiply the input value by 100 before sending it to the API.
Usage
Currency
In some endpoints, you’ll be asked to also specify the currency code. Unless said otherwise, the currency code follows the ISO 4217 standard with three-letter code. For example, "EUR"
, "USD"
, "GBP"
, etc…
JSON Schemas
When working with JSON Schemas, fields asking for a monetary value are identified with the property "inputType": "money"
. Here’s an example of a JSON Schema asking for just one field called signup_bonus_amount
:
1{2 "properties": {3 "signup_bonus_amount": {4 "title": "Signup bonus amount",5 "type": "integer",6 "x-jsf-presentation": {7 "currency": "EUR",8 "inputType": "money"9 }10 }11 }12}
5000
, it means 50.00€ (fifty euros), not five thousand euros — Remember to multiply by 100!1{2 "signup_bonus_amount": 5000 // This means "50.00 EUR" not "5000 EUR" 🚨3}
Minimum / maximum validations
Some fields may come with validations. The example below says the minimum annual gross salary is 2320800
which converting it to an input value means €23,208.00.
1{2 "properties": {3 "annual_gross_salary": {4 "title": "Annual gross salary",5 "type": "integer",6 "minimum": 2320800,7 "x-jsf-presentation": {8 "currency": "EUR",9 "inputType": "money"10 },11 "x-jsf-errorMessage": {12 "minimum": "By law, €23,208.00 is the national minimum wage in Netherlands."13 }14 }15 }16}
Additionally, the field includes the keyword x-jsf-errorMessage
which contains a friendly error message. Note that this keyword is not native in JSON Schema. It’s used by json-schema-form
library to show that error message when necessary. Check the JSF Playground to see it in action.
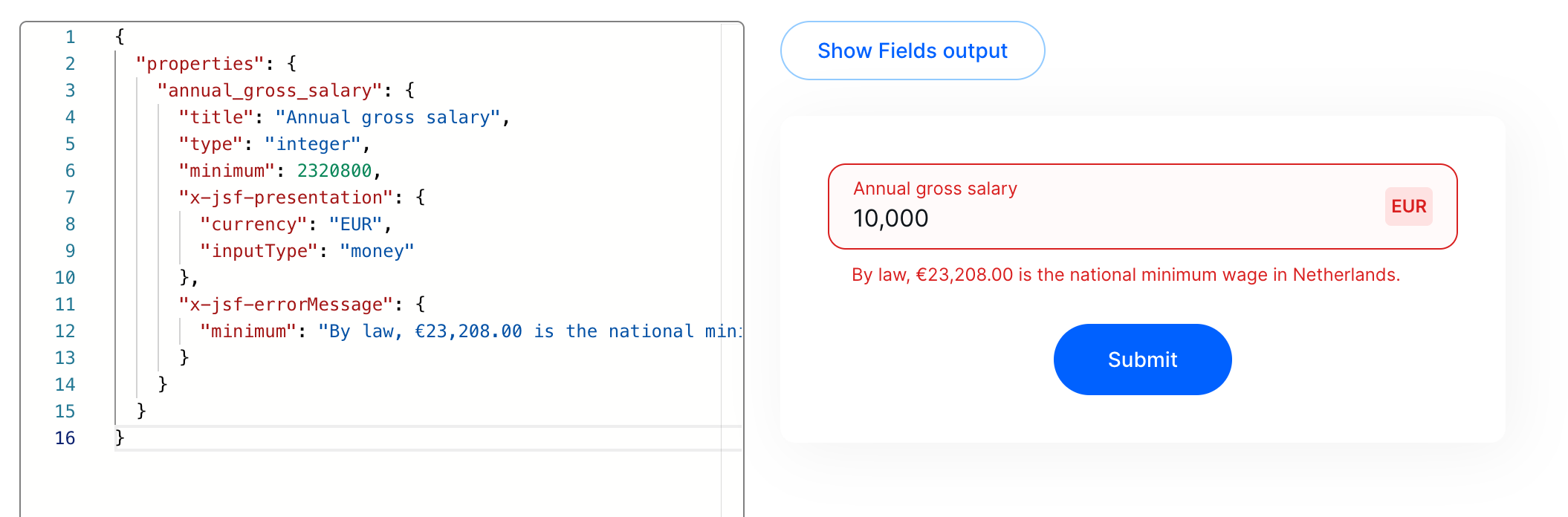
Identifying monetary fields
A monetary field can easily be identified by checking its inputType: "money"
.
If you use json-schema-form
, you can access it in the returned fields
.
1import { createHeadlessForm } from '@remoteoss/json-schema-form';23const { fields } = createHeadlessForm(jsonSchema)45fields.map(field) => {6 console.log(field.inputType)7}
Frontend caveats with Forms
When you build your UI Form, always remember the following things, regardless if you use the json-schema-form
library or not.
- Before sending an input value to the API, multiply the input value by 100. Otherwise, you’ll be sending values 100x smaller than expected.
- When pre-filling an input from the API, always divide the API value by 100. Otherwise, you’ll be showing an input with a value 100x bigger than expected.
- We highly recommend you have strong automated tests in your Money fields, otherwise, you might be sending incorrect values. Imagine paying a salary 100 smaller than expected! 😱