Onboarding an employee
Overview
In this guide, you will learn how to:
- Create an employment
- Send employment data
- Advance the employment through the onboarding process
- Invite the new hire
đź“’Â Table of Contents
Before you get started
Before you get started, ensure that you:
- Are familiar with how to create an employment. If you’re not familiar with how to create an employment, you can follow this guide to create your first company and first employment.
- Have received company consent to act on their behalf. You will need a company-scoped access token to create and manage employments.
Creating your employment
Checking if your desired country is supported
You can check which countries are supported by the Remote API using the list countries endpoint:
1$ curl --location \2 --request GET \3 --header 'Authorization: Bearer eyJraWQ...' \4 'https://gateway.remote-sandbox.com/eor/v1/countries'
https://gateway.remote.com
.If your desired country is not supported, you can reach out to your Remote contact or email api-support@remote.com to let us know of your interest to hire in your country of choice.
Starting with the Basic Information
First, you will need to provide the employment “basic information”. The Remote API asks for this information for every supported country. As the payload might be different from country to country, you will have to look up the expected payload.
Using JSON Schemas for the expected payload
The Remote API uses JSON schemas to define the expected basic information payload. You can use the show form schema endpoint to get the employment_basic_information
JSON schema for your country of choice. Looking at the API documentation, you can see that there are two parameters expected, country_code
and form
:
1GET https://gateway.remote-sandbox.com/v1/countries/{country_code}/{form}
The employment in this guide is located in Canada, so you have to use Canada’s {country_code}
to make this request. The {form}
will be employment_basic_information
.
To get the country_code
of a country, make a request to the supported countries endpoint as shown above. You’ll see that the country_code
for Canada is CAN
.
You’re now ready to make the request:
1$ curl --location \2 --header 'Authorization: Bearer eyJraWQiO...' \3 --request GET 'https://gateway.remote-sandbox.com/v1/countries/CAN/employment_basic_information'
You can then use the resulting JSON Schema to know which fields are required, their labels, descriptions, and validation rules.
Basic Information constraints
Understanding provisional_start_date
validations
Although JSON Schemas have validation rules for each field, the field provisional_start_date
does not include all the validation rules because some are too complex to be described in a JSON schema. As such, some validations are only available through the API, when calling the create employment endpoint. The JSON Schema for this field looks similar to the following:
1{2 "title": "Provisional start date",3 "format": "date",4 "x-jsf-presentation": {5 "inputType": "date",6 "meta": { "mot": 3 },7 "minDate": "2023-09-23"8 }9 }10}
Here’s an explanation for each of those validations:
- Minimum Onboarding Time (MOT): For every supported country, there's a minimum amount of working days Remote needs to effectively onboard an employee before their start date. For example, if today is Sept 19 and the MOT is 3 days, then the selected date needs to be Sept 23 or after. This is included in the JSON Schema as above.
- Weekends: In some countries, the start date cannot be on a weekend. Additionally, weekends are not the same worldwide. For example, some countries consider Sunday a working day. This is not included in the JSON Schema yet.
- Holidays: In some countries, the start date cannot be on a national holiday. This is not included in the JSON Schema yet.
- Arbitrary days: In some countries, certain days are not allowed. For example, the day before Christmas or New Year’s Eve. This is not included in the JSON Schema yet.
The Remote API validates all the rules above per country. If you send a POST or PATCH to /employments
with a date that doesn’t comply with all the rules above, the API will return an error, explaining the reason behind it.
As a visual example, this is how the field looks like in the Remote Platform.
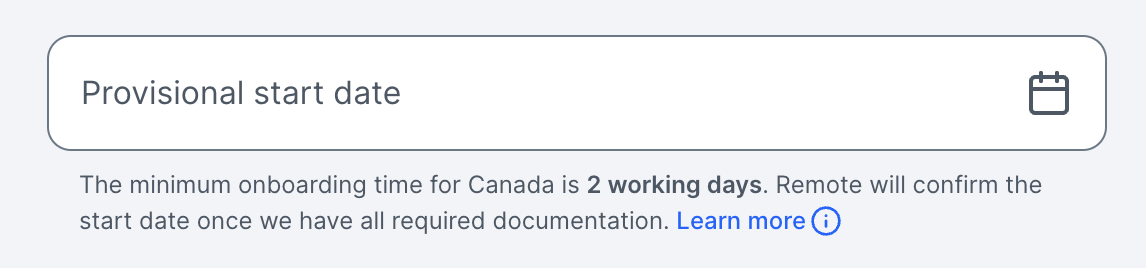
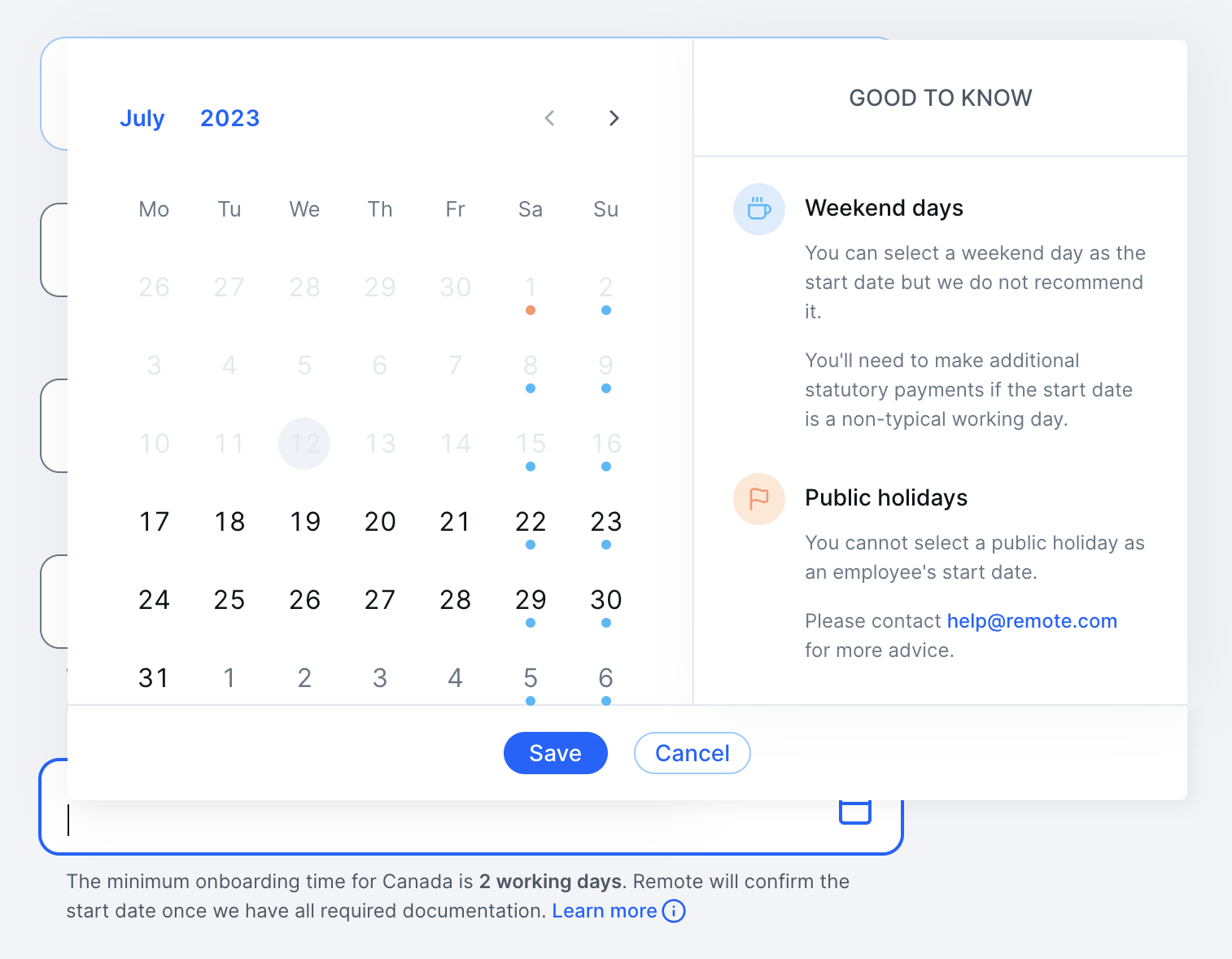
UI enhancements: (coming soon to JSON Schemas). The following features are not included in the JSON Schema. We plan to include it until Q4 2023.
- The “Learn more” text in the description.
- The additional text “Good to Know”
provisional_start_date
validations. Remote plans to add all the validations described above to the JSON Schema in Q4 2023.Validation flow: It’s common for the Customer to take multiple days to finish the Employee Onboarding flow. You can use the meta.mot
number to implement an “expiration system” that notifies your Customer if the start date is no longer valid. For example: Imagine the MOT is 2 days, today is Monday and they selected the start date for Friday. The days have passed and now it’s Thursday but the customer hasn’t finished the flow yet. The start date is no longer valid, so could automatically notify your Customer about it.
Sending the Basic Information to Remote
With the JSON schema describing the basic information, you can submit the data using the create employment endpoint.
Start by sending a POST
request to https://gateway.remote-sandbox.com/v1/employments
. The employment you create will be assigned to the company-scoped access token of the company you would like to create this employment. Here’s how you can build the request:
- Include the
Authorization
header with a valid company-scoped access token. - Include the
Content-Type: application/json
header to indicate the format of the submitted data. - Send the required fields encoded in JSON format. Ensure that the
country_code
you’re using is a supported country using the list countries endpoint.
The basic information data should be sent through the basic_information object:
1$ curl --location \2 --request POST 'https://gateway.remote-sandbox.com/v1/employments' \3 --header 'Authorization: Bearer eyJraWQiO...' \4 --header 'Content-Type: application/json' \5 --data-raw '{6 "country_code": "CAN",7 "basic_information": {8 "name": "Bob Remote",9 "job_title": "Senior Graphic Designer",10 "email": "bob.remote@example.com",11 "provisional_start_date": "2023-05-05",12 "has_seniority_date": "no"13 },14 "type": "employee"15 }'
Being deprecated: Currently, you can also send the basic information data using the root fields, but that’s will soon be deprecated in favor of basic_information
.
1$ curl --location \2 --request POST 'https://gateway.remote-sandbox.com/v1/employments' \3 --header 'Authorization: Bearer eyJraWQiO...' \4 --header 'Content-Type: application/json' \5 --data-raw '{6 "country_code": "CAN",7 "type": "employee",8 // vvv BEING DEPRECATED vvv9 "full_name": "Bob Remote",10 "job_title": "Senior Graphic Designer",11 "personal_email": "bob.remote@example.com",12 "provisional_start_date": "2023-05-05",13 }'
The basic_information object also includes some dynamic fields depending on the country, such as has_seniority_date
. That’s why using JSON Schemas will be crucial here, to ensure each country has its own dynamic required data.
Email tips:
- For the email parameter, in the Sandbox environment, we recommend setting an email address that is unique and can receive emails, so that you can verify that the employee receives the email as you’d expect:
- If you’re using a GMail/GSuite or Outlook email address, you can append custom text to your email address with +randomtext
to make it unique, but still go to the same inbox. For example, if your company email is bob@example.com
, you could do bob+1@example.com
to make it a unique email address, but still have all emails go to your inbox. This is a useful email testing strategy.
Unauthorized
response if it’s expired.Parsing the response
(Click to expand) Here’s an example response you will receive
1{2 "data": {3 "employment": {4 "basic_information": {5 "name": "Bob Remote",6 "job_title": "Senior Graphic Designer",7 "email": "bob.remote@example.com",8 "provisional_start_date": "2023-05-05",9 "has_seniority_date": "no",10 }11 "company_id": "9e88cdac-4e57-46ca-a5a8-580150935cd8",12 "country_code": "CAN",13 "created_at": "2023-02-16T07:30:55",14 "employment_lifecycle_stage": "employment_creation",15 "id": "9fb23136-bb7c-488a-b5dc-37d3b7c9033b",16 "type": "employee",17 "updated_at": "2023-02-16T07:30:56"18 }19 }20}
The employment was created successfully. Most of the response you receive is equivalent to the data you provided, except for one: employment_lifecycle_stage
. This field will be important for moving this employment through the different stages of employment. You can read more about the individual stages here. We will also make use of employment_lifecycle_stage
throughout this guide.
Now that we have an employment, we can get started with its onboarding. Take note of the employment ID, 9fb23136-bb7c-488a-b5dc-37d3b7c9033b
, we’re going to need it later.
Providing employment details
Before the person you’re hiring can be invited to Remote, you will need to provide some information about this employment.
When you create an employment, its employment_lifecycle_stage
is "employment_creation"
. We want to get to the next stage, which is employment_self_enrollment
. The update employment endpoint accepts diverse employment-specific data in its request body.
You’ll need to provide the following details before the next stage to invite the employee:
-
contract_details
pricing_plan_details
The API documentation gives you details on what’s needed for these two fields. For example, the contract_details
:
Contract information. As its fields vary depending on the country, you must query the Show form schema endpoint passing the country code and contract_details as path parameters.
Fetching the necessary JSON schemas
In the same way we did above to fetch the JSON Schemas for the employment_basic_information
, we’ll do the same for contract_details
.
You’re now ready to make the request:
1$ curl --location \2 --request GET 'https://gateway.remote-sandbox.com/v1/countries/CAN/contract_details' \3 --header 'Authorization: Bearer eyJraWQiO...' \
You’ll receive a JSON schema as a result, similar to how we did above with the basic information.
Make sure to do the same for pricing_plan_details
.
Updating the employment with the necessary details
With the contract_details
and pricing_plan_details
JSON Schemas handy, you’re ready to call the update employment endpoint to set the necessary details.
In order to update the employment, you will need to send a PATCH
request to /v1/employments/{id}
. Replace the {id}
with the ID you got for this employment during the employment creation step (for the employment created in this guide, it was 9fb23136-bb7c-488a-b5dc-37d3b7c9033b
):
- Include the
Authorization
header with a valid company-scoped access token. - Include the
Content-Type: application/json
header to indicate the format of the submitted data. - Send the fields you’d like to update encoded in JSON format.
Request example
Here’s an example of what details you may be setting for your employment. Keep in mind that every country has a different fields and they change over time, so yours might look different than the example here:
Example
1$ curl --location --request PATCH 'https://gateway.remote-sandbox.com//v1/employments/9fb23136-bb7c-488a-b5dc-37d3b7c9033b??actions=no_invite' \2 --header 'Authorization: Bearer eyJhbGci...' \3 --header 'Content-Type: application/json' \4 --data-raw '{5 "contract_details": {6 "available_pto": 33,7 "annual_gross_salary": 100000,8 "benefits": {9 "employee_assistance_program": "no",10 "health": "Basic - Employee Only (Canada Life - Basic Health Employee Only; Canada Life - Basic Dental Employee Only; Canada Life - Basic Vision; Canada Life - Basic Life; Canada Life - Basic AD&D)",11 "retirement": "Basic Retirement (Canada Life - Basic Retirement)"12 },13 "bonus_details": "every year",14 "commissions_details": "15% every year",15 "contract_duration": "Permanent",16 "company_business_description": "We are a consulting company, helping businesses implement the best APIs out there.",17 "experience_level": "Level 3 - Associate - Employees who perform independently tasks and/or with coordination and control functions",18 "equity_compensation": {19 "offer_equity_compensation": "no"20 },21 "has_bonus": "yes",22 "has_commissions": "yes",23 "has_signing_bonus": "yes",24 "probation_length": 3,25 "role_description": "Manage a quickly growing and business-critical team. Contribute to hiring and retaining product designers; help to shape the team culture.",26 "province_of_residency": "AB",27 "signing_bonus_amount": 15000,28 "supervisor_name": "Sally",29 "work_address_is_home_address": "yes"30 },31 "pricing_plan_details": {32 "frequency": "annually"33 }34}'
A successful update will result in a 200 OK
response.
Invitation
When you have sent all the required data, you are ready to invite the employee through the Invite employment endpoint.
1$ curl --location \2 --request POST 'https://gateway.remote-sandbox.com/v1/employments/9fb23136-bb7c-488a-b5dc-37d3b7c9033b/invite' \3 --header 'Authorization: Bearer eyJraWQiO...' \
A successful invite will result in a 200 OK
response. The employee will then receive an email from no-reply@remote-sandbox.com
that looks like the image below. Then they’ll start their own self-enrollment to provide the missing details, such as administrative details, home address, etc.
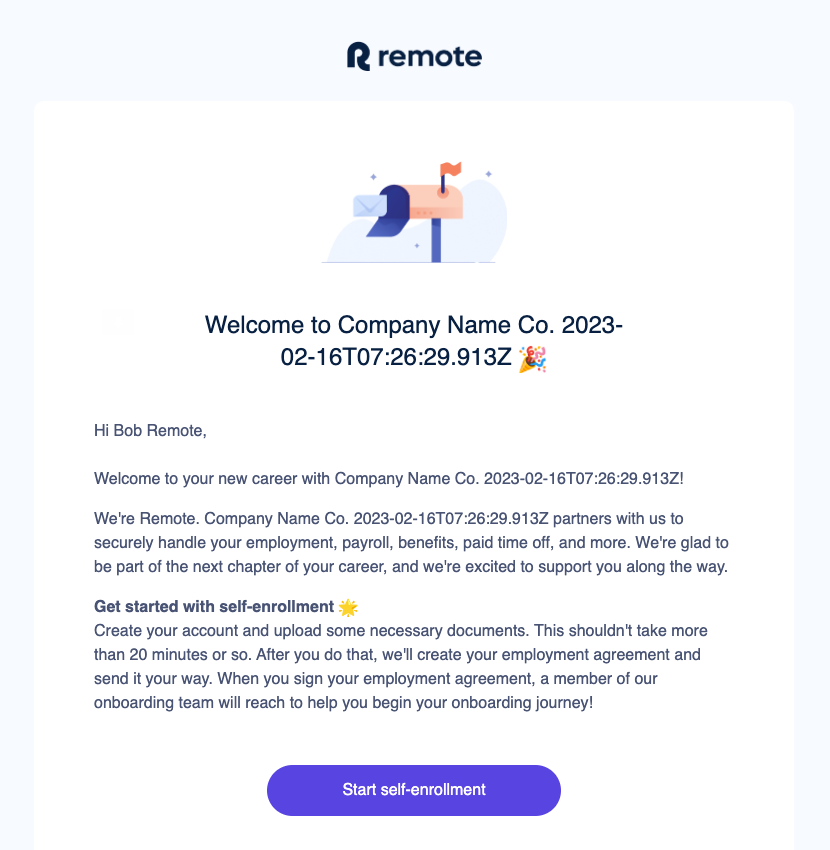
Validations
When this endpoint is called, some data is invalidated to ensure the employment is still valid. For example, the provisional_start_date
is re-validated. If it’s no longer valid, the endpoint will fail and you’ll need to update the invalid data using the Update Employment endpoint.
Checking the employment status
After the invitation, if you need to check again if an employee was already invited, you can look at their employment: If it has employment_lifecycle_stage: "employee_self_enrollment
", it means the employee has received an email to join the Remote platform at their email.
1{2 "data": {3 "employment": {4 ...,5 "employment_lifecycle_stage": "employee_self_enrollment",6 ...,7 "id": "9fb23136-bb7c-488a-b5dc-37d3b7c9033b",8 ...9 "status": "invited",10 }11 }12}
Modifying the employment further
emergency_contact_details
.
If you want to provide additional information for employment, please make sure to do so before the employee is invited. We block updates to the employment data because employees need to agree to amendments in certain cases, such as when there are changes to their contract_details
. Currently, these amendments can only be done through the Remote Platform.It’s possible to change all the information sent during the creation step, as well as add more information to the employment, beyond just the contract and pricing plan details. For example, you can also provide details for home address, bank accounts, emergency contacts, and much more. You can check all possible parameters and details (as they may vary depending on the country of employment) from the Update Employment endpoint documentation.
For example, you can update the emergency contact details for this employment as well:
1$ curl --location \2 --request PATCH 'https://gateway.remote-sandbox.com/v1/employments/9fb23136-bb7c-488a-b5dc-37d3b7c9033b' \3 --header 'Authorization: Bearer eyJraWQiO...' \4 --header 'Content-Type: application/json' \5 --data-raw '{6 "emergency_contact_details": {7 "email": "taylor@example.com",8 "name": "Taylor Johnson",9 "phone_number": "+3519194512312",10 "relationship": "Best friend"11 }12}'
What's next?
Congratulations, you sent the first self-onboarding invitation! You can continue creating and inviting new hires, and also perform other operations such as syncing time off and entering approved expenses. If you have questions regarding the automatic invitation flow, please reach out to the Remote API team at api-support@remote.com
.